Understanding JavaScript Functions: A Comprehensive Guide
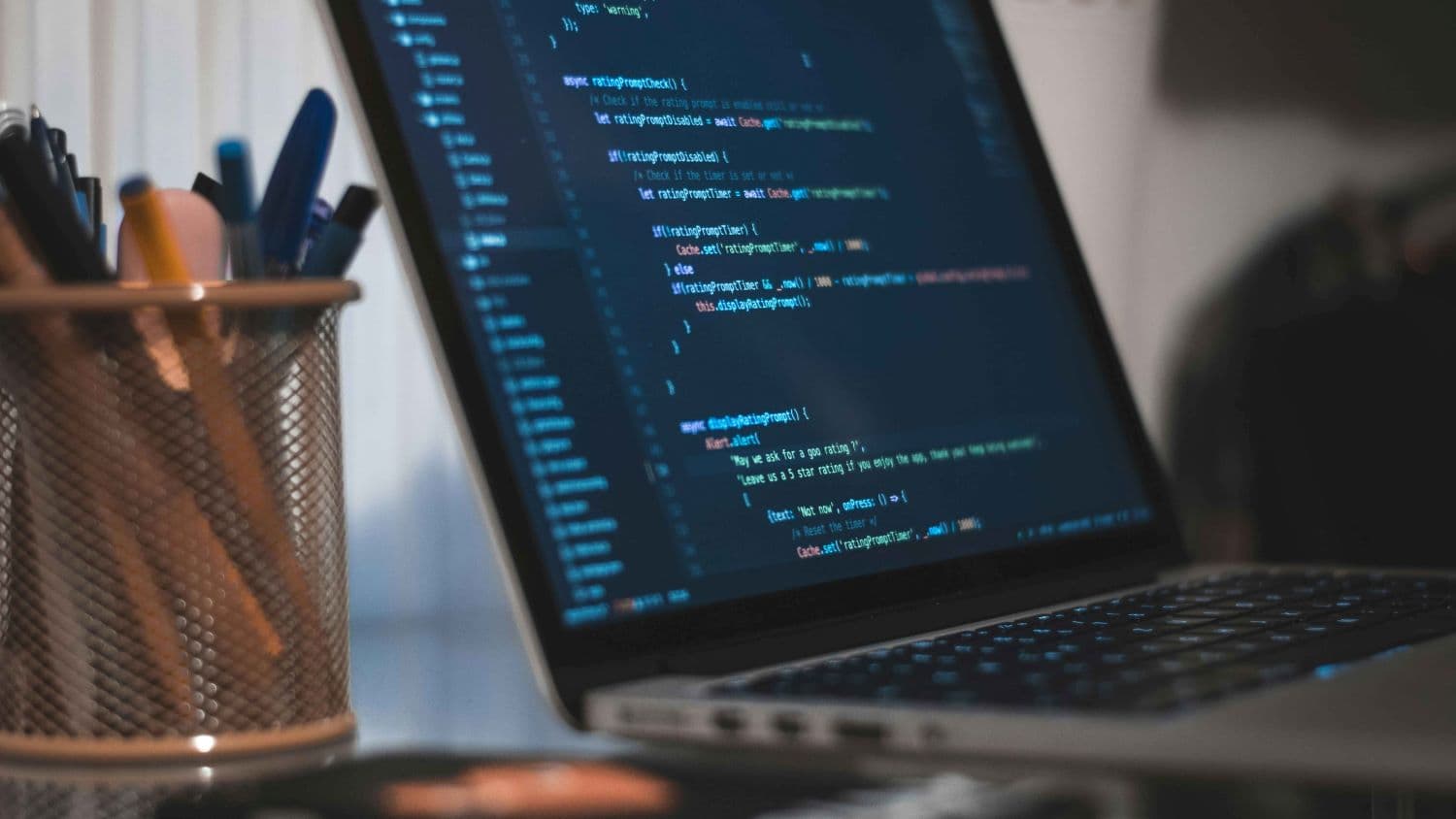
JavaScript functions are essential for efficient coding, whether you're just starting or an experienced developer. In this post, we'll explore their syntax, types, and practical uses to help you write better, maintainable code.
What is a JavaScript Function?
A function in JavaScript is a reusable block of code designed to perform a specific task. Functions allow developers to break down programs into smaller, manageable, and reusable pieces, making code more organized and reducing repetition.
Basic Syntax of a Function
Here’s the basic structure of a JavaScript function:
function functionName(parameters) {
// Code to be executed
return result; // Optional
}
- functionName: The name of the function.
- parameters: Values passed into the function (optional).
- return: Outputs a result from the function (optional).
Example:
function greet(name) {
return "Hello, " + name + "!";
}
console.log(greet("Alice")); // Output: "Hello, Alice!"
Why Use Functions?
- Reusability: Functions help you avoid writing the same code multiple times. Once a function is defined, it can be reused as needed.
- Modularity: Breaking down large tasks into smaller, manageable functions improves code organization.
- Maintenance: Functions make your code easier to maintain. If you need to make changes, you can update a single function without affecting other parts of the code.
- Testing: Functions make it easier to isolate and test specific functionality within your program.
Types of Functions in JavaScript
JavaScript offers multiple ways to define and use functions. Let’s explore the most common types:
1. Function Declarations
A function declaration defines a named function and can be called before it's declared due to JavaScript's hoisting mechanism.
function add(a, b) {
return a + b;
}
console.log(add(5, 3)); // Output: 8
In this case, the function add can be used before the declaration line because of hoisting.
2. Function Expressions
A function expression creates a function and assigns it to a variable. These functions are not hoisted, so they must be defined before they are called.
const multiply = function (a, b) {
return a * b;
};
console.log(multiply(4, 5)); // Output: 20
Function expressions are particularly useful when you want to treat functions as first-class objects, which JavaScript allows.
3. Arrow Functions
Introduced in ES6, arrow functions provide a shorter syntax for writing functions and handle the this context differently compared to traditional functions.
const greet = (name) => {
return "Hello, " + name + "!";
};
console.log(greet("Bob")); // Output: "Hello, Bob!"
For even simpler functions, you can omit the return keyword if the function body contains only a single statement:
const square = (x) => x * x;
console.log(square(4)); // Output: 16
Arrow functions are ideal for concise code but may not be suitable in all contexts (e.g., when using this or in object methods).
4. Immediately Invoked Function Expressions (IIFE)
An IIFE is a function that is executed immediately after it is defined. These are useful for avoiding polluting the global scope in JavaScript.
(function () {
console.log("This function runs immediately!");
})();
The extra parentheses around the function definition tell the JavaScript engine to treat it as an expression, not a declaration.
5. Anonymous Functions
An anonymous function is a function without a name, typically used in places where a function is passed as an argument or used immediately.
setTimeout(function () {
console.log("This message will appear after 2 seconds.");
}, 2000);
In this case, the anonymous function is passed to setTimeout and executed after a 2-second delay.
Parameters and Arguments
JavaScript functions can take parameters when defined and arguments when invoked.
function sum(a, b) {
return a + b;
}
console.log(sum(10, 20)); // Arguments: 10 and 20
Default Parameters
In ES6, JavaScript introduced default parameters that allow you to set default values for function parameters.
function greet(name = "Guest") {
return "Hello, " + name + "!";
}
console.log(greet()); // Output: "Hello, Guest!"
If no argument is provided, the parameter name defaults to "Guest."
Return Values
Functions can return values using the return statement. If no return statement is present, the function returns undefined.
function subtract(a, b) {
return a - b;
}
const result = subtract(10, 4);
console.log(result); // Output: 6
Higher-Order Functions
JavaScript treats functions as first-class citizens, meaning functions can be stored in variables, passed as arguments, and returned from other functions. Higher-order functions are functions that operate on other functions, either by taking them as arguments or returning them.
Example: Passing a function as an argument
function applyOperation(a, b, operation) {
return operation(a, b);
}
const add = (x, y) => x + y;
const result = applyOperation(5, 3, add);
console.log(result); // Output: 8
In this case, the add function is passed as an argument to applyOperation.
Example: Returning a function
function multiplier(factor) {
return function (x) {
return x * factor;
};
}
const double = multiplier(2);
console.log(double(5)); // Output: 10
Here, multiplier returns a new function based on the factor passed to it.
Recursion
Recursion is a technique where a function calls itself to solve smaller instances of the same problem.
Example: Factorial calculation using recursion
function factorial(n) {
if (n === 1) {
return 1;
}
return n * factorial(n - 1);
}
console.log(factorial(5)); // Output: 120
Recursion is useful for tasks that can be broken down into similar subtasks, like tree traversal or mathematical sequences.
Conclusion
Functions in JavaScript are versatile and powerful tools that help you organize, reuse, and maintain your code more effectively. From basic function declarations to higher-order functions and recursion, mastering the different types of functions is essential for any JavaScript developer.
As you dive deeper into JavaScript, experiment with different function types and see how they can simplify your code. Keep practicing, and soon you'll be writing cleaner, more efficient JavaScript programs with ease!
Happy coding! 😃