Date, Time, and Number Formatting in JavaScript: Everything You Need to Know
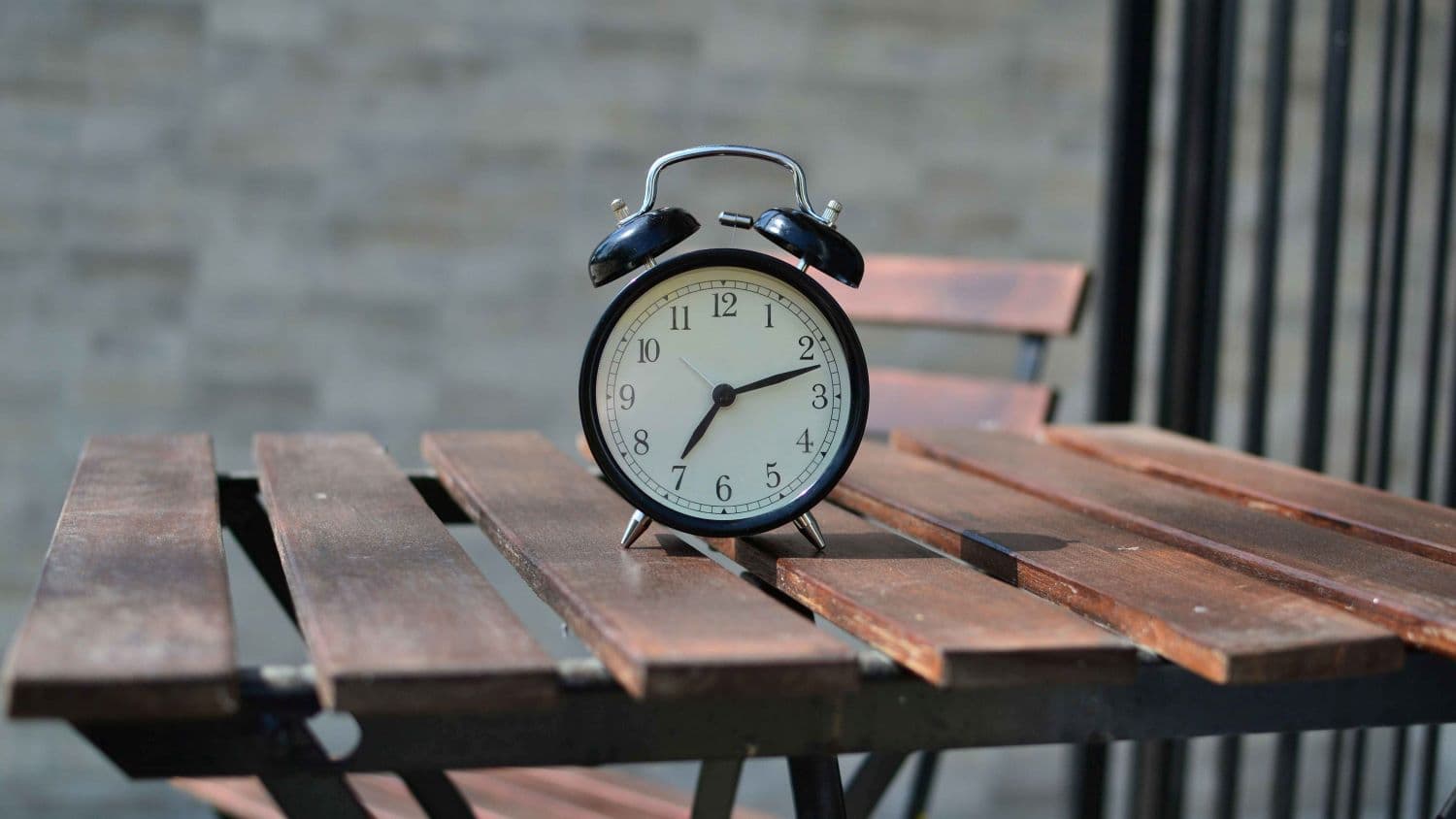
JavaScript provides a robust API for working with dates, times, and numbers, allowing developers to handle time-sensitive applications, perform accurate calculations, and format output for user-friendly interfaces. In this post, we'll dive into handling dates and times with JavaScript's Date object and the Intl.NumberFormat for numbers.
1. JavaScript Date and Time Basics
The Date object in JavaScript is versatile and powerful for handling dates and times. It stores date and time information, and its methods allow easy retrieval, manipulation, and formatting.
Creating a Date Object
There are several ways to create a date object in JavaScript:
// Current date and time
const currentDate = new Date(); // 2024-10-27T05:51:58.818Z
The new Date() returns a ISO 8601 formatted timestamp of current date and time separated by "T"
You can also manipulate this return value by passing date-time string or values into the Date object, for example
// 1. Specified date
const specifiedDate = new Date('2023-10-27'); //2070-10-27T00:00:00.000Z
const specifiedDate=new Date("Decembar 24, 2000"); //2000-12-24T00:00:00.000Z
// 2. Using individual date components
const dateWithComponents = new Date(2002, 9, 19, 10, 23,5); // 2002-10-19T10:23:05.000Z
//💡 Note: month is zero-based
you can create a Date object by passing the number of milliseconds since the Unix Epoch, which is January 1, 1970, 00:00:00 UTC
const currentDate = new Date(1698388320000); //2023-10-27T06:32:00.000Z
Retrieving Date Components
The Date object provides various methods to retrieve parts of the date:
const now = new Date();
console.log(now.getFullYear()); // 2023
console.log(now.getMonth()); // 9 (October, because months are 0-based)
console.log(now.getDate()); // Day of the month (27)
console.log(now.getDay()); // Day of the week (0 for Sunday, 6 for Saturday)
console.log(now.getHours()); // Hours (24-hour format)
console.log(now.getMinutes()); // Minutes
console.log(now.getSeconds()); // Seconds
Modifying Date Components
You can modify date components using corresponding setter methods, which can be helpful for setting specific dates or times.
const date = new Date();
date.setFullYear(2025);
date.setMonth(11); // December (remember months are 0-based)
date.setDate(25);
console.log(date.toString()); // Thu Dec 25 2025 12:02:52 GMT+0530 (India Standard Time)
console.log(date.toLocaleString()); // 12/25/2025, 12:04:05 PM
console.log(date.toTimeString()); // 12:04:39 GMT+0530 (India Standard Time)
console.log(date.toDateString()); // Thu Dec 25 2025
console.log(date.toLocaleDateString()); // 12/25/2025
Date Math
you can get the time step of the current date (milliseconds passed from UNIX time)
const today=new Date()
console.log(today.getTime()); //1730010056845
// OR
console.log(Date.now()) //1730010450418
You can also add or subtract days, hours, or minutes to a date by converting it to milliseconds (the base unit for dates in JavaScript):
const today = new Date();
const tomorrow = new Date(today.getTime() + 24 * 60 * 60 * 1000); // Adding 1 day
console.log(tomorrow);
You also can subtract between two different dates in JavaScript
const timeDiff = new Date(2037, 3, 14) - new Date(2035, 3, 24);
console.log(timeDiff); // 62294400000
2. Formatting Dates and Times
Formatting dates can be done manually or using Intl.DateTimeFormat, which is a much more efficient way of handling dates for various locales.
Using Intl.DateTimeFormat
const now = new Date();
const usFormat = new Intl.DateTimeFormat('en-US').format(now);
const frFormat = new Intl.DateTimeFormat('fr-FR').format(now);
console.log(usFormat); // MM/DD/YYYY for the US
console.log(frFormat); // DD/MM/YYYY for France
Customizing Date Formats
You can customize the output of Intl.DateTimeFormat to show specific parts of a date:
const now=new Date()
const options = {
 hour: 'numeric', //2-digit,
 minute: '2-digit', // numeric
 day: 'numeric', // 2-digit
 weekday: 'long', // narrow , short
 month: 'long', // short , narrow , numeric , 2-digit,
 year: 'numeric', // 2-digit
}
const formattedDate = new Intl.DateTimeFormat('en-US', options).format(now);
console.log(formattedDate); // "Sunday, October 27, 2024 at 12:19 PM"
3. Working with Numbers in JavaScript
JavaScript has the Number type, which can handle integers and floating-point numbers. JavaScript also provides Intl.NumberFormat to manage number formatting, making it easier to handle currency, percentages, and large numbers.
Basic Number Formatting
You can format numbers with Intl.NumberFormat to include commas, decimal points, or different locales.
const number = 1234567.89;
// Default formatting
console.log(new Intl.NumberFormat().format(number)); // "1,234,567.89"
// Locale-specific formatting
console.log(new Intl.NumberFormat('de-DE').format(number)); // "1.234.567,89"
Formatting Currencies
Formatting numbers as currency can be easily handled with Intl.NumberFormat.
const amount = 99.99;
const usOptions = {
 style: 'currency', // currency ,percent ,decimal ,unit
 currency: 'USD', // EUR , YPY , INR  .... all currency
};
const usCurrency = new Intl.NumberFormat('en-US', usOptions).format(amount);
const jpCurrency = new Intl.NumberFormat('ja-JP', { style: 'currency', currency: 'JPY' }).format(amount);
console.log(usCurrency); // "$99.99"
console.log(jpCurrency); // "Â¥100" (rounded to nearest whole number, as per JPY rules)
Formatting Percentages
For percentages, set the style to "percent" and JavaScript will automatically multiply the number by 100.
const fraction = 0.75;
const percentage = new Intl.NumberFormat('en-US', { style: 'percent' }).format(fraction);
console.log(percentage); // "75%"
4. Useful Libraries for Date, Time, and Number Manipulation
Although JavaScript’s built-in Date object and Intl methods are powerful, there are popular libraries that simplify date manipulation and formatting:
- date-fns: A lightweight library with extensive date manipulation functions.
- Moment.js: Although deprecated, it’s still widely used and has great time zone support with moment-timezone.
- Luxon: Modern alternative to Moment.js, built on top of Intl.DateTimeFormat.
Conclusion
Working with dates, times, and numbers in JavaScript can be tricky, especially with locale-specific formatting and precision requirements. Thankfully, JavaScript’s Intl API offers flexible formatting options for both dates and numbers, making it easier to deliver a localized experience. For complex applications, consider using libraries like date-fns or Luxon to handle advanced date manipulations.
Happy coding! ⌚